これまでの記事で、Pythonを使って、音声認識、音声合成、機械翻訳のやり方を説明してきました。今回はこれらの応用として、Pythonを自動翻訳(自動音声翻訳)の実装を解説していきたいと思います。
ここで言う自動翻訳とは、音声で入力されたデータを音声認識にてテキストデータに変換し、それを機械翻訳にて翻訳したものを音声合成にて音声として出力するものです。
それでは早速始めていきましょう。また、これまでの音声認識、音声合成、機械学習に関するぼくの記事を読まれていない方は、以下のリンクから参照して下さい。
はじめに
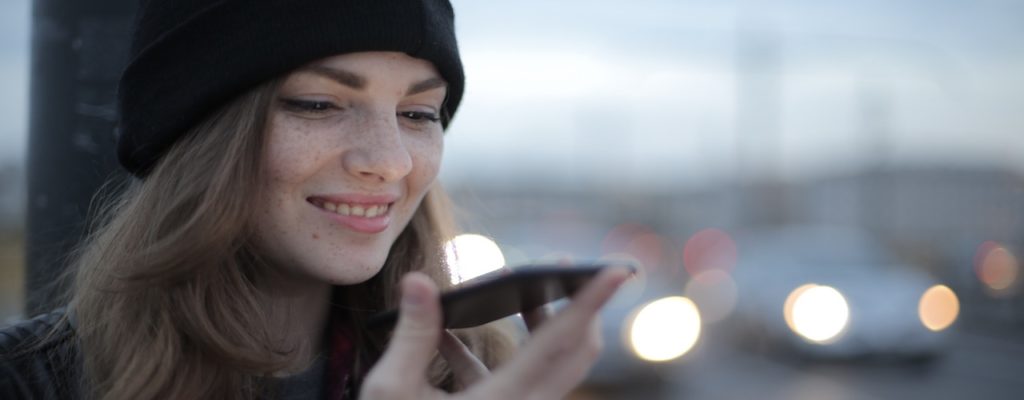
この記事を読んで、できるようになる事・理解できる事
Mac上(Windows上でも大丈夫のはず。)でPythonを使って、音声にて入力した日本語を音声認識、機械翻訳を得て、英文に翻訳し、それを音声合成にて音声に変換し直し、スピーカーにて再生します。
そのためのコードも公開しますので、英語から日本語、また、パラメーターを調整することで、フランス語から中国語等多言語への翻訳にも対応できます。
この記事を読んでほしい方、想定対象者
今回ご紹介するシステムでは、タイムラグ等もあるため、正直なところ、本格的な実用目的での使用には改良が必要かと思います
従いまして、本記事の想定読者には、機械翻訳、機械学習に興味があり、実際のコードの実装を行いたい方、または、機械学習関連の子どもを対象とした教育を行っており、そのための適当な教材を探しておられる方などを、想定しています。
事前に読んでほしい記事・関連記事
本記事は上記の記事で説明したことを一連の動きに合体しています。ただ、まずは本記事を読まれて、興味を持たれてから、上記の記事を参照されてももちろん結構です。
ただ、Pythonにあまり詳しくない方は、コードを実装される前には、必ず上記の記事に目を通してください。
コードの実装
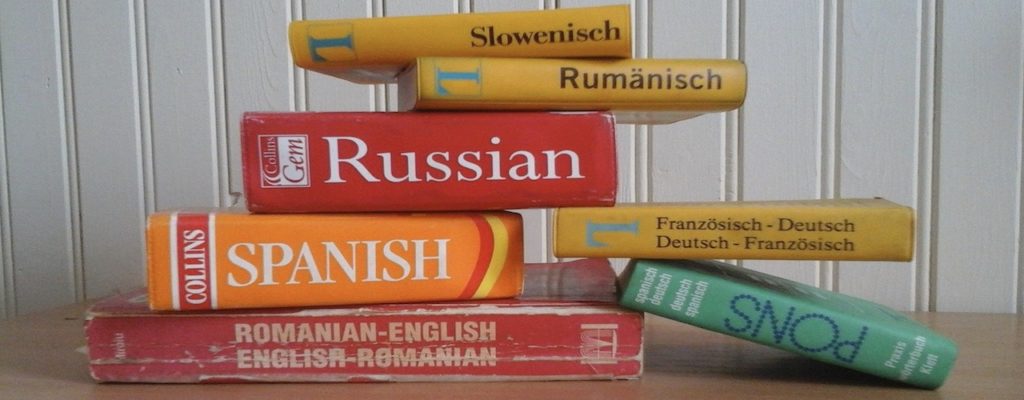
それでは、実際のコードを書いていきましょう。まず初めに今回使うライブラリーをインストールする必要があります。
また、以下のコードはJupyter LabやJupyter Notebookからの入力を想定しています。ターミナルから直接入力する場合は、各行の頭の"!"を省略して下さい。
1 2 3 4 |
# SpeechRecognitionのinstall !pip install speechrecognition # pyaudioのinstall !pip install Pyaudio |
上記で音声認識に必要なライブラリーのインストールを行います。
1 2 |
# pyttsx3のinstall !pip install pyttsx3 |
上記で音声合成に必要なライブラリーのインストールを行います。
1 2 |
# install googletrans !pip install googletrans |
上記で翻訳に必要なライブラリーのインストールと行います。
以下のコマンドを実行してすでにインストールされているライブラリーの一覧を出力して、今回インストールしてライブラリーが入っているか確認しましょう。
1 2 |
# installの確認 !pip list |
それでは、本体コードを以下に示します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
#import speech_recognition as sr import subprocess import tempfile import pyttsx3 from googletrans import Translator # 音声合成 def TextToSpeech_pyttsx3(ph): engine = pyttsx3.init() voices = engine.getProperty('voices') engine.setProperty("voice", voices[18].id) engine.say(ph) engine.runAndWait() # 翻訳 def Translate_ja_to_en(word): # from googletrans import Translator translator = Translator() word2 = translator.translate(word, src='ja', dest='en') text = word2.text return text # 音声入力 while True: r = sr.Recognizer() with sr.Microphone() as source: print("何かお話しして下さい。") audio = r.listen(source) try: # Google Web Speech APIで音声認識 result = r.recognize_google(audio, language="ja-JP") except sr.UnknownValueError: print("Google Web Speech APIは音声を認識できませんでした。") except sr.RequestError as e: print("GoogleWeb Speech APIに音声認識を要求できませんでした;" " {0}".format(e)) else: # word1 = input("翻訳する言葉を入力して!") print(result) word1 = result word2 = Translate_ja_to_en(word1) print(word2) TextToSpeech_pyttsx3(word2) print("--------------------") if result == "終わり": break print("完了。") |
ここでは、ループで質問を繰り返し、「終わり」という音声入力によりループから抜け出し、処理を終えます。また、実行にはインターネットの接続環境が必要です。
言語の選択方法
それでは、それぞれのプロセスでの言語の選択方法について、簡単に説明します。
入力音声言語の選択
具体的には、本体コードの39行目の変数"language"の値で選択しています。コードでの値は"ja-JP"ですが、初めの"ja"は日本語を、次の"JP"は日本国を表します。
つまり、一例として、"en-US"はアメリカで話される英語を、"en-GB"はイギリスで話される英語を示します。
これらの記号は数が多く、ここでの記述には適しませんので、以下のリンクを参照して下さい。
Google Cloud Speech-to-Text 言語サポート
翻訳言語の選択
翻訳言語の選択はコードの23行で行なっています。変数"src"の値が入力言語、"dest"の値が出力言語を表します。
ここで用いる言語とコードの一覧表は以下のリンクから参照できます。
出力音声言語の選択
出力音声の言語は言語単位でももちろんできますが、ばくは声(Voice.id)にて選択する事をお勧めします。以下にそれの一覧表を示します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 |
id No.:0 <Voice id=com.apple.speech.synthesis.voice.Alex name=Alex languages=['en_US'] gender=VoiceGenderMale age=35> id No.:1 <Voice id=com.apple.speech.synthesis.voice.alice name=Alice languages=['it_IT'] gender=VoiceGenderFemale age=35> id No.:2 <Voice id=com.apple.speech.synthesis.voice.alva name=Alva languages=['sv_SE'] gender=VoiceGenderFemale age=35> id No.:3 <Voice id=com.apple.speech.synthesis.voice.amelie name=Amelie languages=['fr_CA'] gender=VoiceGenderFemale age=35> id No.:4 <Voice id=com.apple.speech.synthesis.voice.anna name=Anna languages=['de_DE'] gender=VoiceGenderFemale age=35> id No.:5 <Voice id=com.apple.speech.synthesis.voice.carmit name=Carmit languages=['he_IL'] gender=VoiceGenderFemale age=35> id No.:6 <Voice id=com.apple.speech.synthesis.voice.damayanti name=Damayanti languages=['id_ID'] gender=VoiceGenderFemale age=35> id No.:7 <Voice id=com.apple.speech.synthesis.voice.daniel name=Daniel languages=['en_GB'] gender=VoiceGenderMale age=35> id No.:8 <Voice id=com.apple.speech.synthesis.voice.diego name=Diego languages=['es_AR'] gender=VoiceGenderMale age=35> id No.:9 <Voice id=com.apple.speech.synthesis.voice.ellen name=Ellen languages=['nl_BE'] gender=VoiceGenderFemale age=35> id No.:10 <Voice id=com.apple.speech.synthesis.voice.fiona name=Fiona languages=['en-scotland'] gender=VoiceGenderFemale age=35> id No.:11 <Voice id=com.apple.speech.synthesis.voice.Fred name=Fred languages=['en_US'] gender=VoiceGenderMale age=30> id No.:12 <Voice id=com.apple.speech.synthesis.voice.ioana name=Ioana languages=['ro_RO'] gender=VoiceGenderFemale age=35> id No.:13 <Voice id=com.apple.speech.synthesis.voice.joana name=Joana languages=['pt_PT'] gender=VoiceGenderFemale age=35> id No.:14 <Voice id=com.apple.speech.synthesis.voice.jorge name=Jorge languages=['es_ES'] gender=VoiceGenderMale age=35> id No.:15 <Voice id=com.apple.speech.synthesis.voice.juan name=Juan languages=['es_MX'] gender=VoiceGenderMale age=35> id No.:16 <Voice id=com.apple.speech.synthesis.voice.kanya name=Kanya languages=['th_TH'] gender=VoiceGenderFemale age=35> id No.:17 <Voice id=com.apple.speech.synthesis.voice.karen name=Karen languages=['en_AU'] gender=VoiceGenderFemale age=35> id No.:18 <Voice id=com.apple.speech.synthesis.voice.kyoko.premium name=Kyoko languages=['ja_JP'] gender=VoiceGenderFemale age=35> id No.:19 <Voice id=com.apple.speech.synthesis.voice.laura name=Laura languages=['sk_SK'] gender=VoiceGenderFemale age=35> id No.:20 <Voice id=com.apple.speech.synthesis.voice.lekha name=Lekha languages=['hi_IN'] gender=VoiceGenderFemale age=35> id No.:21 <Voice id=com.apple.speech.synthesis.voice.luca name=Luca languages=['it_IT'] gender=VoiceGenderMale age=35> id No.:22 <Voice id=com.apple.speech.synthesis.voice.luciana name=Luciana languages=['pt_BR'] gender=VoiceGenderFemale age=35> id No.:23 <Voice id=com.apple.speech.synthesis.voice.maged name=Maged languages=['ar_SA'] gender=VoiceGenderMale age=35> id No.:24 <Voice id=com.apple.speech.synthesis.voice.mariska name=Mariska languages=['hu_HU'] gender=VoiceGenderFemale age=35> id No.:25 <Voice id=com.apple.speech.synthesis.voice.mei-jia name=Mei-Jia languages=['zh_TW'] gender=VoiceGenderFemale age=35> id No.:26 <Voice id=com.apple.speech.synthesis.voice.melina name=Melina languages=['el_GR'] gender=VoiceGenderFemale age=35> id No.:27 <Voice id=com.apple.speech.synthesis.voice.milena name=Milena languages=['ru_RU'] gender=VoiceGenderFemale age=35> id No.:28 <Voice id=com.apple.speech.synthesis.voice.moira name=Moira languages=['en_IE'] gender=VoiceGenderFemale age=35> id No.:29 <Voice id=com.apple.speech.synthesis.voice.monica name=Monica languages=['es_ES'] gender=VoiceGenderFemale age=35> id No.:30 <Voice id=com.apple.speech.synthesis.voice.nora name=Nora languages=['nb_NO'] gender=VoiceGenderFemale age=35> id No.:31 <Voice id=com.apple.speech.synthesis.voice.paulina name=Paulina languages=['es_MX'] gender=VoiceGenderFemale age=35> id No.:32 <Voice id=com.apple.speech.synthesis.voice.samantha name=Samantha languages=['en_US'] gender=VoiceGenderFemale age=35> id No.:33 <Voice id=com.apple.speech.synthesis.voice.sara name=Sara languages=['da_DK'] gender=VoiceGenderFemale age=35> id No.:34 <Voice id=com.apple.speech.synthesis.voice.satu name=Satu languages=['fi_FI'] gender=VoiceGenderFemale age=35> id No.:35 <Voice id=com.apple.speech.synthesis.voice.sin-ji name=Sin-ji languages=['zh_HK'] gender=VoiceGenderFemale age=35> id No.:36 <Voice id=com.apple.speech.synthesis.voice.tessa name=Tessa languages=['en_ZA'] gender=VoiceGenderFemale age=35> id No.:37 <Voice id=com.apple.speech.synthesis.voice.thomas name=Thomas languages=['fr_FR'] gender=VoiceGenderMale age=35> id No.:38 <Voice id=com.apple.speech.synthesis.voice.ting-ting name=Ting-Ting languages=['zh_CN'] gender=VoiceGenderFemale age=35> id No.:39 <Voice id=com.apple.speech.synthesis.voice.veena name=Veena languages=['en_IN'] gender=VoiceGenderFemale age=35> id No.:40 <Voice id=com.apple.speech.synthesis.voice.Victoria name=Victoria languages=['en_US'] gender=VoiceGenderFemale age=35> id No.:41 <Voice id=com.apple.speech.synthesis.voice.xander name=Xander languages=['nl_NL'] gender=VoiceGenderMale age=35> id No.:42 <Voice id=com.apple.speech.synthesis.voice.yelda name=Yelda languages=['tr_TR'] gender=VoiceGenderFemale age=35> id No.:43 <Voice id=com.apple.speech.synthesis.voice.yuna name=Yuna languages=['ko_KR'] gender=VoiceGenderFemale age=35> id No.:44 <Voice id=com.apple.speech.synthesis.voice.yuri name=Yuri languages=['ru_RU'] gender=VoiceGenderMale age=35> id No.:45 <Voice id=com.apple.speech.synthesis.voice.zosia name=Zosia languages=['pl_PL'] gender=VoiceGenderFemale age=35> id No.:46 <Voice id=com.apple.speech.synthesis.voice.zuzana name=Zuzana languages=['cs_CZ'] gender=VoiceGenderFemale age=35> |
これらは、コードの15行目のvoices[ ]のカギカッコの中の数字で指定しています。上のコードでは、18が指定されており、以下の声(name=Kyoko)が選択されています。
1 2 3 4 5 6 |
id No.:18 <Voice id=com.apple.speech.synthesis.voice.kyoko.premium name=Kyoko languages=['ja_JP'] gender=VoiceGenderFemale age=35> |
言語のコードは入力音声言語の選択でご紹介したリンクから探していただき、それに会う声を選択して下さい。
まとめ
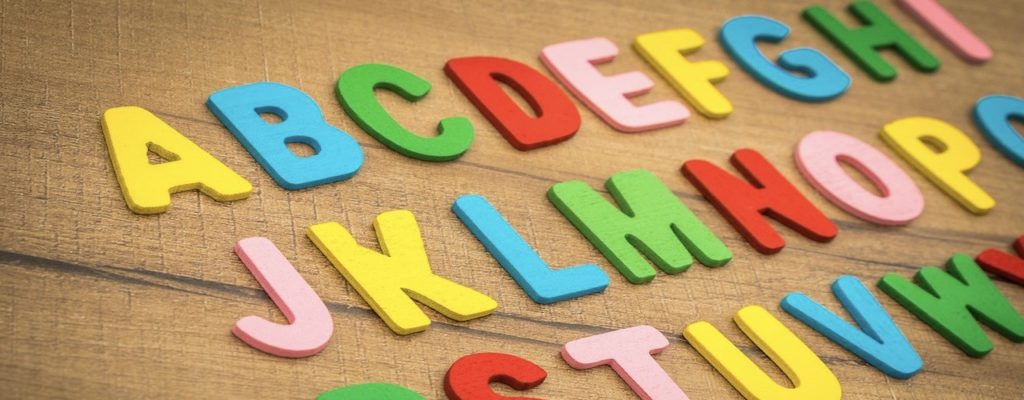
Pythonの長所の一つに強力なライブラリーを数多く持っていることが挙げられますが、今回はそれを実感していただけたのではないでしょうか。
音声認識、翻訳、音声合成という複雑な機能をこんなにも簡単なコードで実装できることは、一昔前ならば、考えられないことだと思います。
今回ご紹介した以外にも、もっとたくさんのライブラリーがPythonには用意されています。機会があれば、また、ご紹介できればと思います。
それでは最後までお付き合いいただき、ありがとうございました。さようなら。
コメント